How to load a ragdoll into the correct state?
I check the work like that.
I start the scene frame by frame.
1) activate the ragdoll and save it
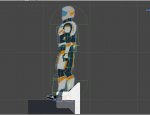
2) simulate a drop a few frames ahead
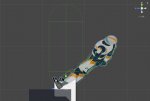
3) load the saved state of the ragdoll
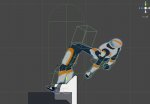
all colliders for some reason in the wrong places
I check the work like that.
I start the scene frame by frame.
1) activate the ragdoll and save it
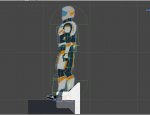
2) simulate a drop a few frames ahead
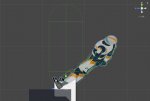
3) load the saved state of the ragdoll
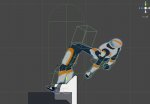
all colliders for some reason in the wrong places
C#:
public override void Save(JObject jObject)
{
base.Save(jObject);
if (IsActive)
{
var jrbs = new JArray();
for (int i = 0; i < m_Rigidbodies.Length; ++i)
{
var rb = m_Rigidbodies[i];
var jEntity = new JObject();
jEntity.Add("pos", rb.position.GetAsString());
jEntity.Add("rot", rb.rotation.GetAsString());
jEntity.Add("vel", rb.velocity.GetAsString());
jEntity.Add("avel", rb.angularVelocity.GetAsString());
jrbs.Add(jEntity);
}
jObject.Add("rbs", jrbs);
}
}
public override void Load(JObject jObject)
{
base.Load(jObject);
var prop = jObject.Property("rbs");
if (prop != null)
{
m_Force = Vector3.zero;
m_Position = Vector3.zero;
m_CharacterLocomotion.TryStartAbility(this, true, true);
var jrbs = (JArray)prop.Value;
var index = 0;
foreach (var rbInfo in jrbs)
{
var rb = m_Rigidbodies[index];
var jEntity = (JObject)rbInfo;
rb.position = SerializationUtils.Vector3Parse((string)jEntity.GetValue("pos"));
rb.rotation = SerializationUtils.QuaternionParse((string)jEntity.GetValue("rot"));
rb.velocity = SerializationUtils.Vector3Parse((string)jEntity.GetValue("vel"));
rb.angularVelocity = SerializationUtils.Vector3Parse((string)jEntity.GetValue("avel"));
index++;
}
}
}