zach.fransen
New member
My general objective is to have a behavior where a group of enemies self-organizes into a formation and patrols multiple waypoints.
Now, I did accomplish this, but I'm thinking the way I approached it may not have been ideal.
I combined the Patrol task from the movement pack with the column task from the formations pack. I went ahead and had these run in parallel, with some modifications.
I neutered the patrol class to have it set a Vector3 shared variable instead of setting destination via the navmeshagent. I also modified the FormationGroup class to have it so that it doesn't end when the formation reaches the destination. I then had the formation task reference the Vector3 set by the patrol task in order to have the crew move around appropriately and the results seem reasonably good. I feel comfortable inheriting from the patrol task instead of modifying it directly, and I can override the appropriate method in my code. However, I don't see a great way to influence the FormationGroup.cs logic without having to override each individual formation or I can yank out a lot of distance calculation and coordination logic and make my own custom formation task, but that's not awesome.
Feel free to tell me what I did was silly and there's a configuration option I missed or some other suggestion.
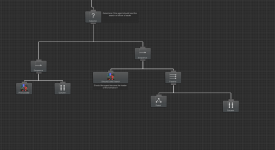
Now, I did accomplish this, but I'm thinking the way I approached it may not have been ideal.
I combined the Patrol task from the movement pack with the column task from the formations pack. I went ahead and had these run in parallel, with some modifications.
I neutered the patrol class to have it set a Vector3 shared variable instead of setting destination via the navmeshagent. I also modified the FormationGroup class to have it so that it doesn't end when the formation reaches the destination. I then had the formation task reference the Vector3 set by the patrol task in order to have the crew move around appropriately and the results seem reasonably good. I feel comfortable inheriting from the patrol task instead of modifying it directly, and I can override the appropriate method in my code. However, I don't see a great way to influence the FormationGroup.cs logic without having to override each individual formation or I can yank out a lot of distance calculation and coordination logic and make my own custom formation task, but that's not awesome.
Feel free to tell me what I did was silly and there's a configuration option I missed or some other suggestion.
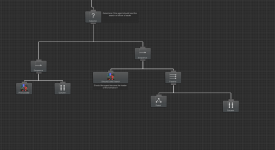