I have a struct defined with nullable fields, and it's used as shared variable like so:
I noticed when a task that has a public SharedNavAgentData field invokes it's OnStart() method, the Value parameter shows all fields of the struct contain a value, which is not what I was expecting.
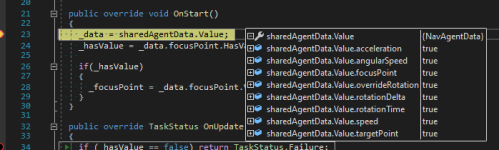
Do shared variables initialize nullables under the hood?
Code:
public struct NavAgentData
{
public Vector3? targetPoint;
public Vector3? focusPoint;
public float? speed;
public float? acceleration;
public float? angularSpeed;
public float? rotationDelta;
public float? rotationTime;
public bool? overrideRotation;
}
[System.Serializable]
public class SharedNavAgentData : SharedVariable<NavAgentData>
{
public static implicit operator SharedNavAgentData(NavAgentData value)
{
return new SharedNavAgentData { Value = value };
}
}
I noticed when a task that has a public SharedNavAgentData field invokes it's OnStart() method, the Value parameter shows all fields of the struct contain a value, which is not what I was expecting.
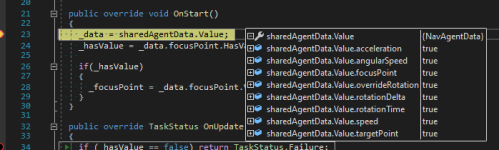
Do shared variables initialize nullables under the hood?