I have a patrol task, which is running and don't give a chance to other tasks to work with the visible target within line of sight. I need to break patrol, if AI sees target
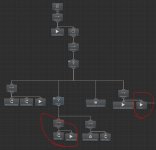
C#:
///Patrol
public override TaskStatus OnUpdate() {
if (PatrolPoint.Value == Vector3.zero) {
return TaskStatus.Failure;
}
if (NavMeshAgentDecorator.HasArrived()) {
return TaskStatus.Success;
}
GotoNextPoint();
return TaskStatus.Running;
}
///WithinSight:
public override TaskStatus OnUpdate() {
for (int i = 0; i < _possibleTargets.Length; i++) {
var possibleTarget = _possibleTargets[i];
if (possibleTarget == null) {
continue;
}
Vector3 startRayPos;
Vector3 direction;
if (!IsWithinSight(possibleTarget, out startRayPos, out direction))
continue;
float distance;
Ray rayToTarget = RayToTarget(possibleTarget, startRayPos, direction, out distance);
RaycastHit hit;
bool isHitObstacle = Physics.Raycast(rayToTarget, out hit, Mathf.Min(distance, ViewDistance),
LayerMask.GetMask(Obstacles));
if (isHitObstacle)
continue;
Target.Value = possibleTarget;
Debug.Log($"[{possibleTarget.name}] is within sight of [{gameObject.name}]");
return TaskStatus.Success;
}
Target.Value = null;
return TaskStatus.Failure;
}