Cheo
Active member
Hello, I'd like to request two simple additions related to objects spawned on destruction.
First of all, here is something I had already posted 2 months ago - you can see the issue yourself in a few moments by just trying to destroy a crate which scale isn't of 1. https://www.opsive.com/forum/index.php?threads/spawned-objects-on-death-scale-fix.10021/ The fix I shared is very simple and any dev can instantly understand why it needs to be added.
Next, we need to have the option to use either the normal or Quaternion.Identity for objects instantiated in Object Spawn Info. Here's what this class should look like :
A clear example of why this fix is necessary as well is a vfx issue I recently stumbled upon when trying out different vfx explosion prefabs from Hovl's explosion pack for my UCC grenade - here's a screenshot of the explosion when Use Normal is set to false :
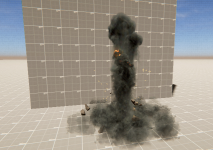
And when it is set to true :
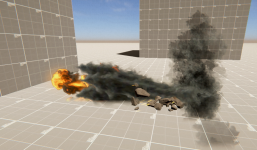
As you can see, this vfx prefab is meant to be shooting upwards, and can't follow the normal axis. Once again, any fellow game dev will instantly see the necessity of this change too.
Hope all this can be included in 3.0.17 so that we don't have to take care of that ourselves. Thanks in advance.
First of all, here is something I had already posted 2 months ago - you can see the issue yourself in a few moments by just trying to destroy a crate which scale isn't of 1. https://www.opsive.com/forum/index.php?threads/spawned-objects-on-death-scale-fix.10021/ The fix I shared is very simple and any dev can instantly understand why it needs to be added.
Next, we need to have the option to use either the normal or Quaternion.Identity for objects instantiated in Object Spawn Info. Here's what this class should look like :
C#:
/// <summary>
/// Represents the object which can be spawned.
/// </summary>
[System.Serializable]
public class ObjectSpawnInfo
{
#pragma warning disable 0649
[Tooltip("The object that can be spawned.")]
[SerializeField] private GameObject m_Object;
[Tooltip("The probability that the object can be spawned.")]
[Range(0, 1)] [SerializeField] private float m_Probability = 1;
[Tooltip("Should a random spin be applied to the object after it has been spawned?")]
[SerializeField] private bool m_RandomSpin;
[SerializeField] private bool m_UseNormal = true;
[SerializeField] private bool m_KeepParentScale = true;
#pragma warning restore 0649
public GameObject Object { get { return m_Object; } }
public float Probability { get { return m_Probability; } }
public bool RandomSpin { get { return m_RandomSpin; } }
public bool UseNormal { get { return m_UseNormal; } }
public bool KeepParentScale { get { return m_KeepParentScale; } }
/// <summary>
/// Instantiate the object.
/// </summary>
/// <param name="position">The position to instantiate the object at.</param>
/// <param name="normal">The normal of the instantiated object.</param>
/// <param name="gravityDirection">The normalized direction of the character's gravity.</param>
/// <returns>The instantiated object (can be null). </returns>
public GameObject Instantiate(Vector3 position, Vector3 normal, Vector3 gravityDirection)
{
if (m_Object == null) {
return null;
}
// There is a random chance that the object cannot be spawned.
if (UnityEngine.Random.value < m_Probability) {
var rotation = Quaternion.LookRotation(normal);
if (!m_UseNormal)
{
rotation = Quaternion.identity;
}
// A random spin can be applied so the rotation isn't the same every hit.
if (m_RandomSpin) {
rotation *= Quaternion.AngleAxis(UnityEngine.Random.Range(0, 360), normal);
}
var instantiatedObject = ObjectPoolBase.Instantiate(m_Object, position, rotation);
// If the DirectionalConstantForce component exists then the gravity direction should be set so the object will move in the correct direction.
var directionalConstantForce = instantiatedObject.GetCachedComponent<Traits.DirectionalConstantForce>();
if (directionalConstantForce != null) {
directionalConstantForce.Direction = gravityDirection;
}
return instantiatedObject;
}
return null;
}
}
A clear example of why this fix is necessary as well is a vfx issue I recently stumbled upon when trying out different vfx explosion prefabs from Hovl's explosion pack for my UCC grenade - here's a screenshot of the explosion when Use Normal is set to false :
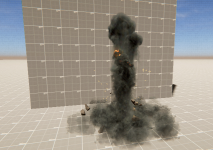
And when it is set to true :
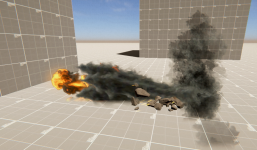
As you can see, this vfx prefab is meant to be shooting upwards, and can't follow the normal axis. Once again, any fellow game dev will instantly see the necessity of this change too.
Hope all this can be included in 3.0.17 so that we don't have to take care of that ourselves. Thanks in advance.