In many cases, it might be necessary to bind both a double click and a single click of the same key to different functions. However, UCC is unable to distinguish between a double click and a single click relative to it.
Below is the method I have added, called GetSinglePress:
Support for the SinglePress Input can also be incorporated within the Ability.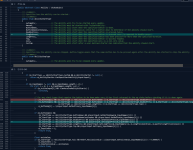
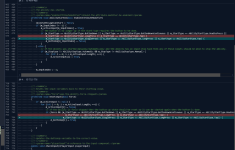
Below is the method I have added, called GetSinglePress:
Code:
/// <summary>
/// Returns true if a double press occurred (double click or double tap).
/// </summary>
/// <param name="buttonName">The button name to check for a double press.</param>
/// <returns>True if a double press occurred (double click or double tap).</returns>
public bool GetDoublePress(string buttonName)
{
if (GetButtonDown(buttonName)) {
if (m_ButtonDownTime == null) {
m_ButtonDownTime = new Dictionary<string, float>();
}
if (m_ButtonDownTime.TryGetValue(buttonName, out var time)) {
if (time != Time.unscaledTime && time + m_DoublePressTapTimeout > Time.unscaledTime)
{
m_ButtonDownTime.Remove(buttonName);
return true;
}
m_ButtonDownTime[buttonName] = Time.unscaledTime;
} else {
m_ButtonDownTime.Add(buttonName, Time.unscaledTime);
}
}
return false;
}
/// <summary>
/// Returns true if a single press occurred (As opposed to double press).
/// </summary>
/// <param name="buttonName">The button name to check for a single press.</param>
/// <returns>True if if a single press occurred (As opposed to double press).</returns>
public bool GetSinglePress(string buttonName)
{
if (m_ButtonDownTime == null)
{
m_ButtonDownTime = new Dictionary<string, float>();
}
if (m_ButtonDownTime.TryGetValue(buttonName, out var time))
{
if (time != Time.unscaledTime && time + m_DoublePressTapTimeout <= Time.unscaledTime)
{
m_ButtonDownTime.Remove(buttonName);
return true;
}
}
return false;
}
Support for the SinglePress Input can also be incorporated within the Ability.
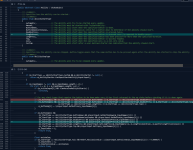
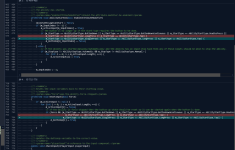