Hi,
I tried the following: For every recipe my Player can get I make an Item which holds a recipe as an attribute.
Before I trigger the crafter I'll find every Item from the category "Crafting Recipe" in the players inventory and then get the Recipe-value of those items. Those i want to add to the recipes of the crafter but somehow it doesn't work.
What am I doing wrong? (Or is there a even a simpler way than this?)
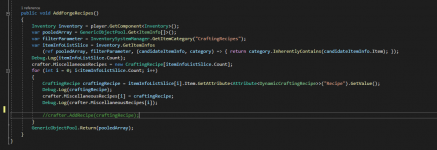
I am puzzled about the two DebugLogs inside the forloop: The first is giving me the name of the craftingRecipe, so it is not null. But when I check the first entry of the MiscellaneousRecipes-array after adding this Recipe it is null.
And indeed, using this method before I open the crafter leads to an empty first field above the items I can craft (the items I added as default recipes to the crafter in the Inspector.)
Thanks for your help
I tried the following: For every recipe my Player can get I make an Item which holds a recipe as an attribute.
Before I trigger the crafter I'll find every Item from the category "Crafting Recipe" in the players inventory and then get the Recipe-value of those items. Those i want to add to the recipes of the crafter but somehow it doesn't work.
What am I doing wrong? (Or is there a even a simpler way than this?)
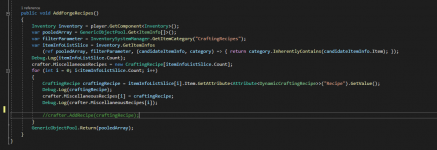
I am puzzled about the two DebugLogs inside the forloop: The first is giving me the name of the craftingRecipe, so it is not null. But when I check the first entry of the MiscellaneousRecipes-array after adding this Recipe it is null.
And indeed, using this method before I open the crafter leads to an empty first field above the items I can craft (the items I added as default recipes to the crafter in the Inspector.)
Thanks for your help