Hey, I'm having difficulty setting up a system of attribute adding/removal.
When equipping an item I want to "add stats".
When removing the item, I want to "remove stats".
When swapping items, I want to "remove original item stats, then replace it with the new items stats".
When dropping, I want to "if Equipped, then remove stats when dropped, else don't remove stats when dropped". Currently it removes stats when UnEquipped since I don't know how to perform a "isEquipped" from the drop item action. (that means value goes negative when no value should change, since it was never equipped)
The code below was a custom solution that doesn't use the Event system. We don't know how to properly implement the feature above. We're just making an attempt to get it working.
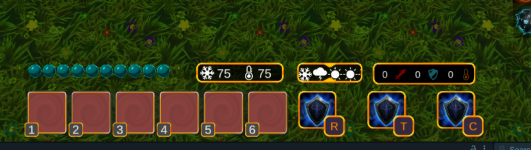
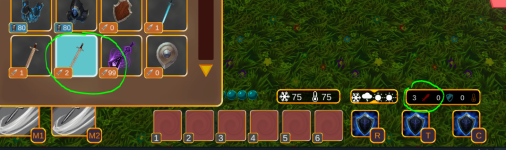
Here is my custom PlayerStatsManager that receives the data and updates the Stats/UI. It interacts with the UIS item action (see next class below). It calls "ApplyAtributeStats" when called through Equip/Unequip item actions.
It cycles through the entire list of attributes and checks for specific strings like "AttackRating, Defense Rating, TemperatureRating", and if any of those are true (multiple can be true) then it executes an Add/Remove of that type. Not the best solution but it seems to work.
When equipping an item I want to "add stats".
When removing the item, I want to "remove stats".
When swapping items, I want to "remove original item stats, then replace it with the new items stats".
When dropping, I want to "if Equipped, then remove stats when dropped, else don't remove stats when dropped". Currently it removes stats when UnEquipped since I don't know how to perform a "isEquipped" from the drop item action. (that means value goes negative when no value should change, since it was never equipped)
The code below was a custom solution that doesn't use the Event system. We don't know how to properly implement the feature above. We're just making an attempt to get it working.
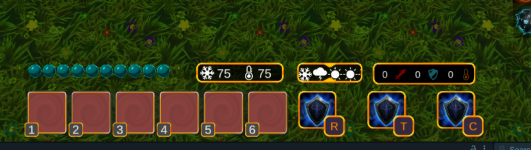
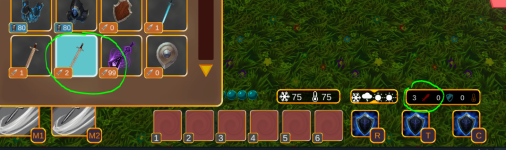
Here is my custom PlayerStatsManager that receives the data and updates the Stats/UI. It interacts with the UIS item action (see next class below). It calls "ApplyAtributeStats" when called through Equip/Unequip item actions.
It cycles through the entire list of attributes and checks for specific strings like "AttackRating, Defense Rating, TemperatureRating", and if any of those are true (multiple can be true) then it executes an Add/Remove of that type. Not the best solution but it seems to work.
C#:
using UnityEngine;
using Item = Opsive.UltimateInventorySystem.Core.Item;
namespace GoblinQuest
{
public class PlayerStatsManager : MonoBehaviour
{
// DESC: CONTAINS ALL PLAYER STATS
public static PlayerStatsManager Instance;
[Header("Armor")]
public int defenseRating;
[Header("Body Temperature")]
public int temperatureRating;
[Header("Attack")]
public int attackRating;
public int attackSpeed;
[Header("Movement Speed")]
public int defaultMoveSpeed;
private void Awake()
{
Instance = this;
}
// ATTRIBUTES CALLED BY ITEM ACTION (SEE "EQUIP/UNEQUIP" OR "DROP" ITEM ACTION)
public void ApplyAttributeStats(Item item, bool isAdding)
{
// Loops through the Item attributes.
var includeItemDefinitionAttributes = true;
var includeItemCategoryAttributes = false;
var attributeCount = item.GetAttributeCount(includeItemDefinitionAttributes, includeItemCategoryAttributes);
for (int i = 0; i < attributeCount; i++)
{
SetAttribute(item, isAdding, i);
}
}
// SETS ATTRIBUTE FOR THE FOR LOOP
private void SetAttribute(Item item, bool isAdding, int i)
{
var attribute = item.GetAttributeAt(i, true, true);
if (attribute.Name == "AttackRating")
{
int attackValue = (int)attribute.GetValueAsObject();
//Debug.Log("Found Attack: " + attackValue);
if (isAdding)
{
//Debug.Log("Added Attack: " + attackValue);
AddAttack(attackValue);
}
else
{
//Debug.Log("Removed Attack: " + attackValue);
RemoveAttack(attackValue);
}
}
if (attribute.Name == "DefenseRating")
{
int attackValue = (int)attribute.GetValueAsObject();
//Debug.Log("Found Attack: " + attackValue);
if (isAdding)
{
//Debug.Log("Added Attack: " + attackValue);
AddDefense(attackValue);
}
else
{
//Debug.Log("Removed Attack: " + attackValue);
RemoveDefense(attackValue);
}
}
if (attribute.Name == "TemperatureRating")
{
int attackValue = (int)attribute.GetValueAsObject();
//Debug.Log("Found Attack: " + attackValue);
if (isAdding)
{
//Debug.Log("Added Attack: " + attackValue);
AddTemperature(attackValue);
}
else
{
//Debug.Log("Removed Attack: " + attackValue);
RemoveTemperature(attackValue);
}
}
}
//
// CALLS FUNCTION BASED ON DATA RECIEVED ABOVE!
//
// BODY TEMPERATURE -> TOO HOT/COLD = DAMAGE!
public void AddTemperature(int temperature)
{
temperatureRating += temperature;
AttackArmorDisplayUI.Instance.UpdateRatingsUI();
}
public void RemoveTemperature(int temperature)
{
temperatureRating -= temperature;
AttackArmorDisplayUI.Instance.UpdateRatingsUI();
}
// ARMOR DEFENSE RATING!
public void AddDefense(int armor)
{
defenseRating += armor;
AttackArmorDisplayUI.Instance.UpdateRatingsUI();
}
public void RemoveDefense(int armor)
{
defenseRating -= armor;
AttackArmorDisplayUI.Instance.UpdateRatingsUI();
}
// ATTACK WEAPON RATING!
public void AddAttack(int attack)
{
attackRating += attack;
AttackArmorDisplayUI.Instance.UpdateRatingsUI();
}
public void RemoveAttack(int attack)
{
attackRating -= attack;
AttackArmorDisplayUI.Instance.UpdateRatingsUI();
}
}
}
Last edited: