cptscrimshaw
Member
Whenever the crafting menu opens, I'm trying to figure out the best way to have the recipe list selected so that I can use the gamepad to move up and down the list. If I hover the mouse over the recipe list, then the gamepad works fine, but I can't get the gamepad to recognize the recipe list when the crafting menu is opened. It appears to be selected, but won't work until I move the mouse first.
Any ideas?
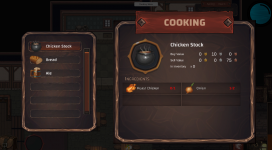
Any ideas?
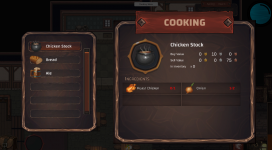